I wasted some time for this so I decided to post the solution. If you have problem returning dynamically drawn image from controller below informations may help.
The task looks easy and should be achieved with this code:
Perceptive person will notice that after writing image to the stream it position property isn't at the beginning and FileStreamResult apparently can't deal with this.
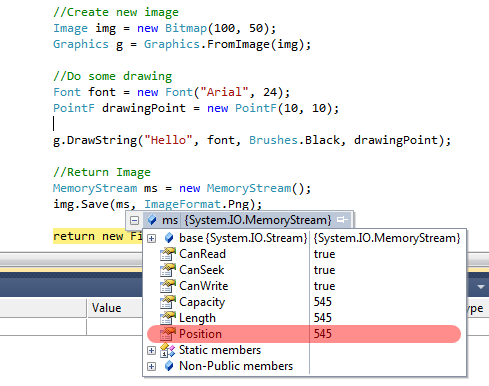
After reseting stream position everything works well (bold line).
The task looks easy and should be achieved with this code:
public ActionResult Hello(string text)Unfortunately this won't work. At least not in .Net 4.0.
{
//Create new image
Image img = new Bitmap(100, 50);
Graphics g = Graphics.FromImage(img);
//Do some drawing
Font font = new Font("Arial", 24);
PointF drawingPoint = new PointF(10, 10);
g.DrawString(text, font, Brushes.Black, drawingPoint);
//Return Image
MemoryStream ms = new MemoryStream();
img.Save(ms, ImageFormat.Png);
return new FileStreamResult(ms, "image/png");
}
Perceptive person will notice that after writing image to the stream it position property isn't at the beginning and FileStreamResult apparently can't deal with this.
After reseting stream position everything works well (bold line).
public ActionResult Hello(string text)Additionally the view which asynchronously (but without ajax) retrieve image from our controller:
{
//Create new image
Image img = new Bitmap(100, 50);
Graphics g = Graphics.FromImage(img);
//Do some drawing
Font font = new Font("Arial", 24);
PointF drawingPoint = new PointF(10, 10);
g.DrawString(text, font, Brushes.Black, drawingPoint);
//Return Image
MemoryStream ms = new MemoryStream();
img.Save(ms, ImageFormat.Png);
ms.Position = 0;
return new FileStreamResult(ms, "image/png");
}
<div id="divResult"></div>
<input type="text" id="txtText" value="Hello" />
<input type="button" name="submit" onclick="javascript:Hello();" value="Draw" />
<script type="text/javascript">
function Hello()
{
var link = '@Url.Content("~/Controller/Hello")';
var txt = document.getElementById('txtText').value;
link += '?';
link += 'text=' + txt;
document.getElementById('divResult').innerHTML = '<img src="' + link + '" alt="' + txt+ '" />';
return false;
}
</script>